In the ever-growing ecosystem of React libraries and frameworks, finding the right tools for building scalable, maintainable applications is essential. After working with Next.js and RTK Query across several projects, I’ve been thrilled with the results: the tech stack has made our code cleaner, faster, and far easier to maintain. With its powerful features like server-side rendering and built-in API routes, I’ve been impressed how well-structured and efficient our React projects can be.
Why Next.js?
Next.js, a React-based framework, provides developers with tools for building optimised, production-ready applications. What sets Next.js apart is its clear, well-defined project structure and its ability to seamlessly integrate server-side rendering (SSR) and static site generation (SSG), in contrast to the traditional client-side rendering (CSR) approach typically used in React apps. One of the major reasons we picked up Next.js framework is its SSR capability, which provides clearly readable code structure and data flow.
Image below shows a basic implementation of Next.js SSR.
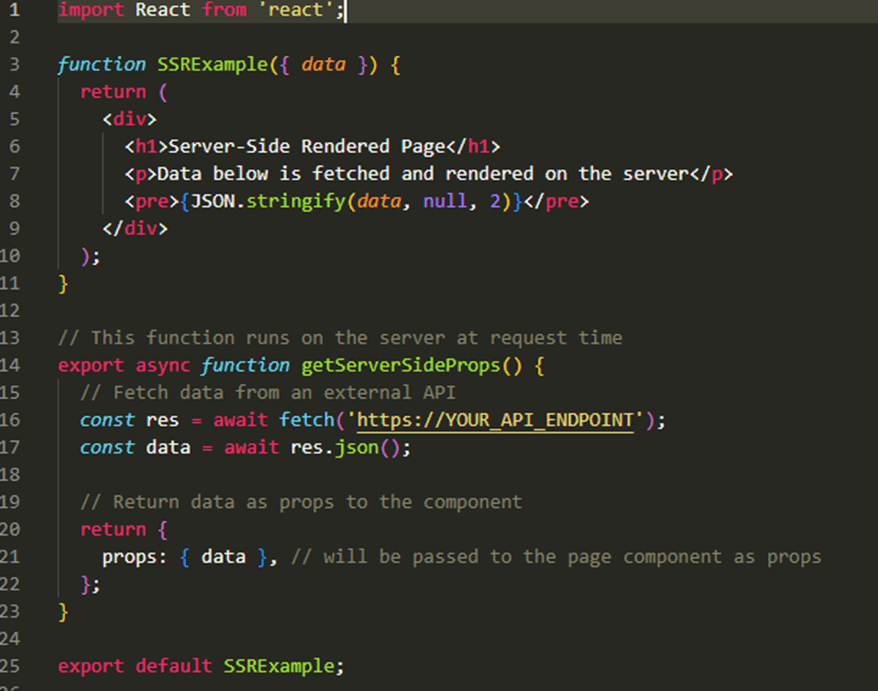
Let me briefly explain the data flow:
- The user requests data from the web page (this could be by using a button or simply by opening the page).
- The application determines that this particular action uses getServerSideProps, which fetches the data from the API.
- After retrieving and processing the data inside getServerSideProps, it is passed back to the component as props.
With this type of implementation, developers won’t need to set up a specific function call in every place that requires data fetching. The data can be fetched in real time, ensuring that users always see the most up-to-date information. Server-rendered content is also easier for search engines to crawl, improving SEO.
Next.js not only optimises load times but also helps streamline development and enhance maintainability. Additionally, API routes allow us to define server-side logic directly within the app, reducing the need for separate backend for simple API needs.
Here’s a basic example of API routes in Next.js
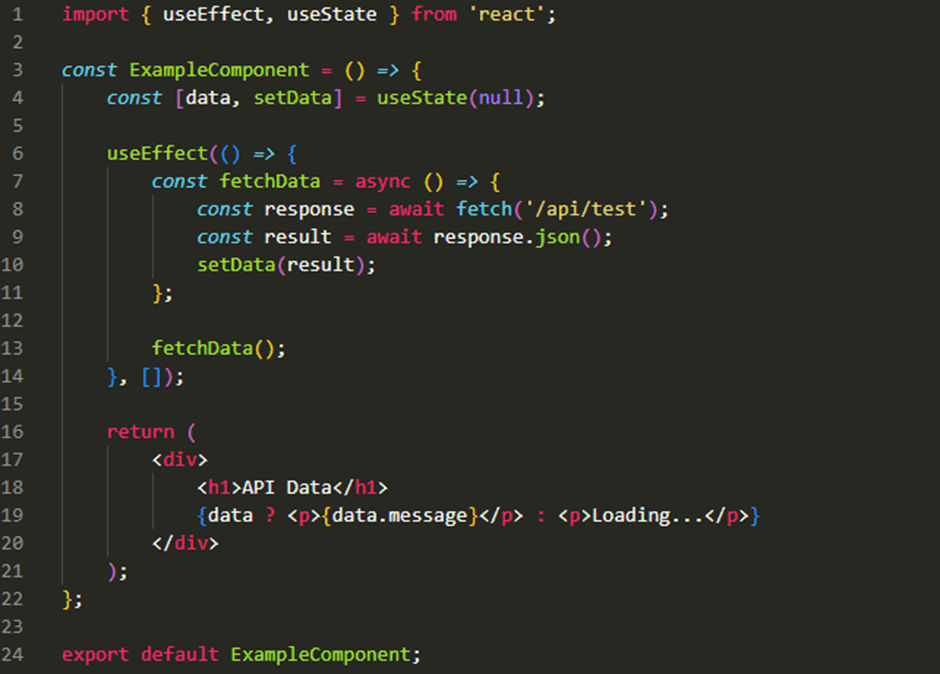
In the ExmapleComponent, I’m fetching data from /api/test route under fetchData() function.
In the test route file, I can define some custom logics about this request. For example, I’m simply saying that in this request, only GET method is allowed. Next.js API routes allow you to create server-side logic directly within your frontend app, this may not be directly linked to business logics, but can streamline the architecture and make the codebase more manageable.
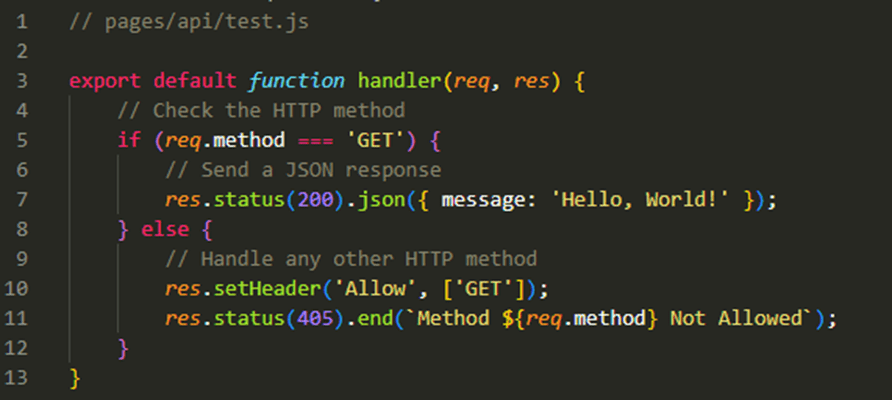
What is RTK Query?
RTK Query is a powerful data-fetching and caching solution that is part of the Redux Toolkit, which itself is built on Redux—a predictable state container for JavaScript applications. Redux helps manage the application state globally, making it easier to share data across components, while the Redux Toolkit simplifies the process of writing Redux logic by reducing boilerplate code and providing useful utilities.
It is specifically designed to streamline API interactions in React applications, allowing developers to focus on building features rather than managing complex state logics. Traditionally, handling asynchronous data in React involves a cumbersome setup with significant boilerplate code. This complexity can quickly become overwhelming, leading to hard-to-maintain code as applications grow.
Benefits of Using RTK Query:
- Centralised API Services: RTK Query allows us to define “endpoints” that represent various API interactions in a structured manner. This means that all our API calls are centralised in one place, making it easier to manage and maintain them, and this can significantly reduce the risk of errors and inconsistencies across the application.
- Simplified State Management: With RTK Query, we can manage loading, error, and success states automatically. It handles caching, deduplication, and re-fetching logic for us, meaning we don’t have to implement this functionality manually. This centralisation of state management simplifies our application’s data flow, making it more predictable.
- Efficiency in Code Maintenance: by automating many aspects of API integration, RTK Query significantly reduces the boilerplate code we would otherwise need to write. Team members can easily understand how data is fetched and manipulated, making onboarding new developers simpler and faster.
- Consistency Across Applications: Using RTK Query helps ensure that our API interactions are consistent across different components and applications. By defining endpoints and caching logic in a unified manner, we reduce the risk of discrepancies that can arise from handling API calls in various ways.
Let me give a quick setup overview of RTK Query. Firstly, you will need to install the tool by NPM:
npm install @reduxjs/toolkit react-redux
Then setting up the Store:
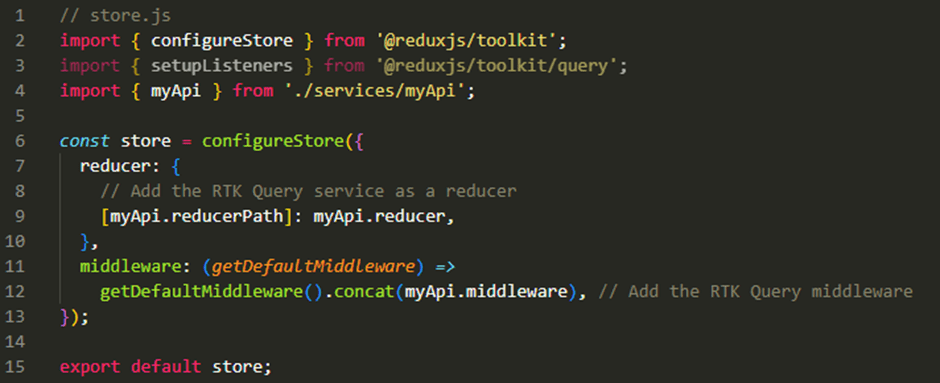
Define an API service using createApi function, and here you can define the actions such as GET, POST, DELETE, PUT:
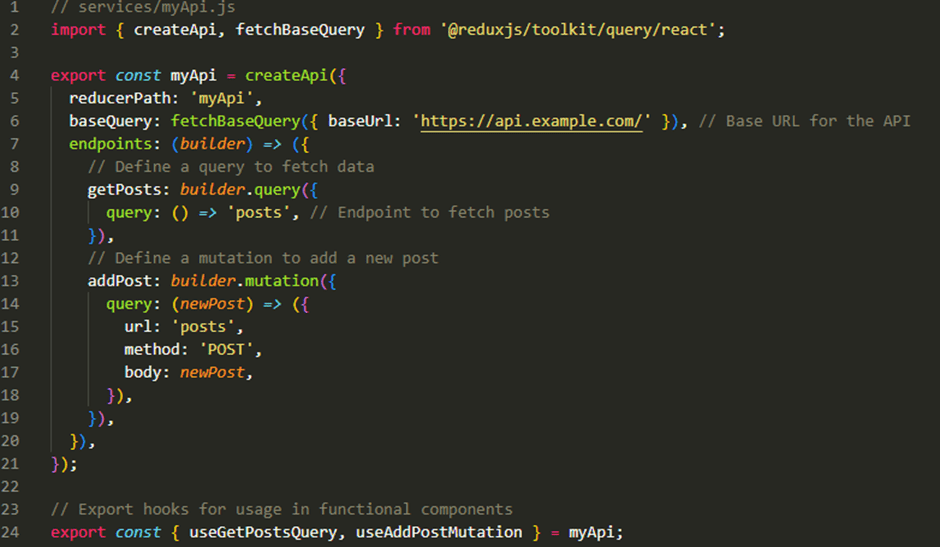
Your API can then be used in components as hooks:
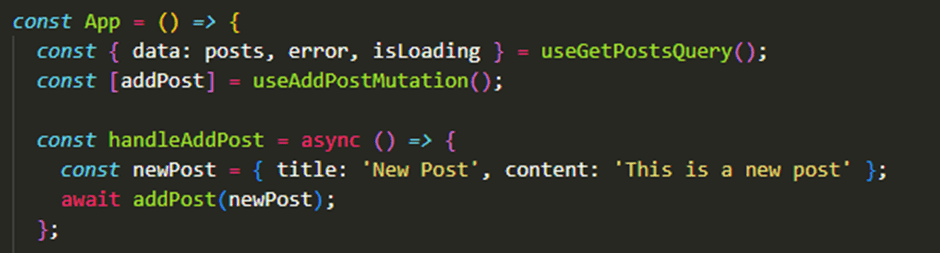
By centralising all API functions, you can ensure that all custom logic related to API fetching is consolidated in one location. This approach makes it easier to trace and debug any issues that may arise during data retrieval.
We’ve chosen Next.js and RTK Query to build scalable, maintainable React applications. With server-side rendering (SSR) and built-in API routes, Next.js boosts performance, while RTK Query centralises API services and simplifies state management. Together, they streamline development and improve the user experience—tools we plan to keep leveraging for future projects.